Lazy loading is a technique that speeds up page loads on web pages. Its idea in all its simplicity is to refrain from loading any images that are not visible. Any images will be loaded just before they appear as the visitor scrolls the page. This should make no apparent difference in the user experience but the loading times will be greatly improved, especially on sites that feature a lot of images.
Native Support for Lazy Loading in Chrome
This optimization has been possible to create with JavaScript already, but what makes the topic relevant today is the fact that Chrome will have native support for it from version 76 onwards. You can take it into use simply by adding the attribute loading="lazy"
to image and iframe elements on your site. On WordPress sites, this can be achieved simply by installing the Native Lazy Load plugin by Google. The plugin will automatically add the attributes for Chrome and also employ a fallback based on Intersection Observer API for other browsers.
Lazy Loading Example
An example on what the HTML looks like before installing the plugin:
<img src="https://seravo.com/wp-content/uploads/2019/06/janine-joles-1143603-unsplash-230x230.jpg">
…and after:
<img src="https://seravo.com/fi/wp-content/plugins/native-lazyload/assets/images/placeholder.svg" loading="lazy" data-src="https://seravo.com/wp-content/uploads/2019/06/janine-joles-1143603-unsplash-230x230.jpg">
In addition to the attribute needed to activate lazy loading, the actual image resource specified data-src
In addition to this, a CSS snippet that hides images in the initial page load is applied in the HTML head.
<style type="text/css">
.no-js .native-lazyload-js-fallback {
display: none;
}
</style>
This piece of JavaScript is added to the end of the page. It takes care of compatibility with other browsers and earlier versions of Chrome:
<script type="text/javascript">
( function() {
var nativeLazyloadInitialize = function() {
var lazyElements, script;
if ( 'loading' in HTMLImageElement.prototype ) {
lazyElements = [].slice.call( document.querySelectorAll( '.native-lazyload-js-fallback' ) );
lazyElements.forEach( function( element ) {
if ( ! element.dataset.src ) {
return;
}
element.src = element.dataset.src;
delete element.dataset.src;
if ( element.dataset.srcset ) {
element.srcset = element.dataset.srcset;
delete element.dataset.srcset;
}
if ( element.dataset.sizes ) {
element.sizes = element.dataset.sizes;
delete element.dataset.sizes;
}
element.classList.remove( 'native-lazyload-js-fallback' );
} );
} else if ( ! document.querySelector( 'script#native-lazyload-fallback' ) ) {
script = document.createElement( 'script' );
script.id = 'native-lazyload-fallback';
script.type = 'text/javascript';
script.src = 'https://seravo.com/fi/wp-content/plugins/native-lazyload/assets/js/lazyload.js';
script.defer = true;
document.body.appendChild( script );
}
};
if ( document.readyState === 'complete' || document.readyState === 'interactive' ) {
nativeLazyloadInitialize();
} else {
window.addEventListener( 'DOMContentLoaded', nativeLazyloadInitialize );
}
}() );
</script>
To get to know the plugin more intimately, you can investigate its source code in GitHub.
The Difference that Lazy Loading Makes
Before the plugin was taken into use the front page of our Finnish site loaded a total of 68 images.
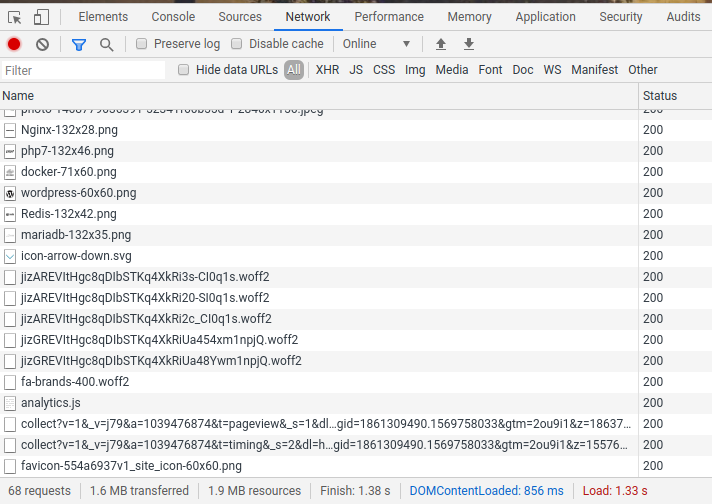
After the plugin was installed the front page loaded only 54 images.
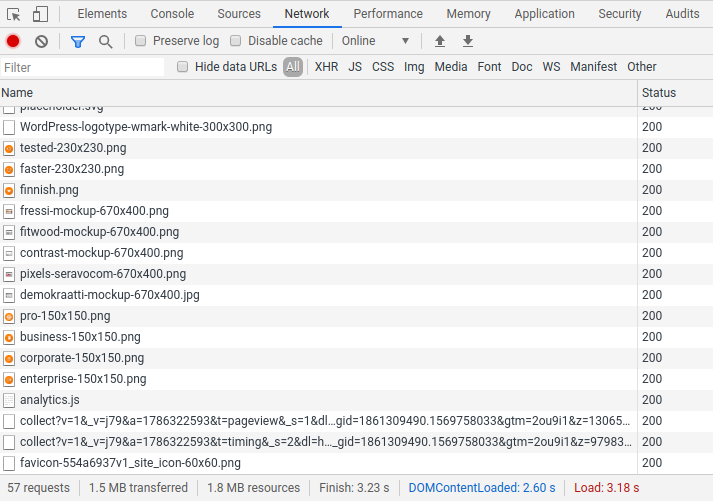
The rest of the images get loaded as the visitor scrolls the page.
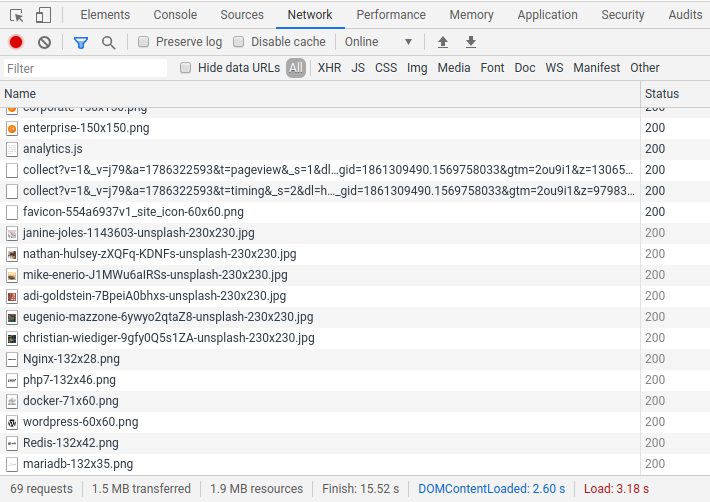
You can also compare the before and after at webpagetest.org.
Conclusion
Using native lazy loading seems to be pretty effortless with these new tools. We are yet to come up with anything that would prevent us from recommending you to give it a try. We are also considering making the Native Lazy Load plugin a part of the Seravo project template by default.
The same functionality can, of course, be coded into a WordPress theme without the help of the plugin. When it comes to browser support, we’re hoping that other manufacturers will follow Google’s lead swiftly to make this optimization native in their browsers.